Python vs Java: Making the Right Choice for Your Software Development Project
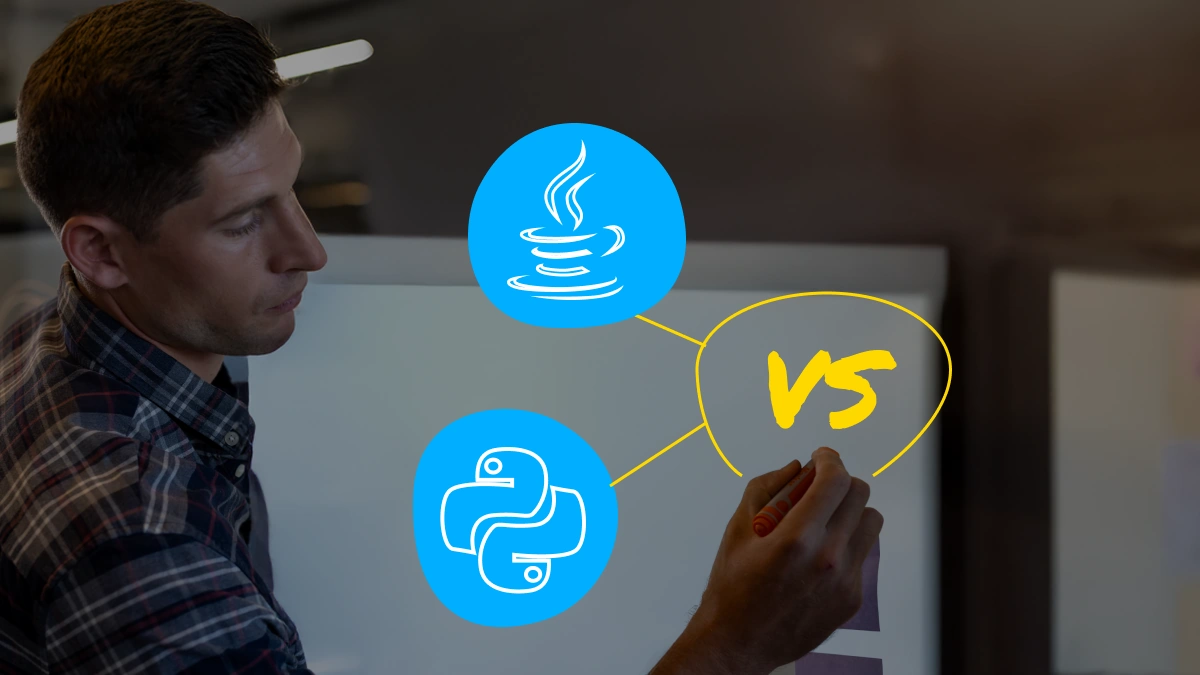
When choosing between Python and Java for your software project, there’s no one-size-fits-all answer. For example, if you’re building a financial analytics dashboard with heavy data processing, Python’s data science libraries might save you weeks of development. But if you’re launching a mobile banking platform with thousands of concurrent users, Java’s performance and thread safety might be your best bet.
In this article, we break down the core technical and business differences between the two languages — based on real use cases, performance benchmarks, and our experience staffing dedicated developers for startups and enterprises worldwide.
Introduction to Python and Java
According to the Stack Overflow 2024 Survey, Python and Java are among the seven most popular programming languages for software solutions used by seasoned software developers during the past year.
What Is Python?
Python has earned its place as one of the most widely used programming languages thanks to its simplicity and flexibility. Many companies choose to hire Python developers when working on projects in data science, AI, web development, automation, or scripting — areas where quick prototyping and fast development really matter. With a clean syntax, cross-platform support, and a rich set of libraries, Python makes it easier to build scalable and adaptable software without overcomplicating the process.
What Is Java?
Java remains a cornerstone of enterprise software development thanks to its strong performance, portability across platforms, and long-term maintainability. It’s the foundation of countless backend systems, Android apps, and large-scale cloud-based platforms — especially where strict type safety and system stability are priorities. With a robust ecosystem of frameworks like Spring and Hibernate, Java helps companies build reliable, scalable solutions that can handle complex business logic and high user loads.
Key Differences Between Java and Python
Java and Python take very different approaches to software development, from how they handle types and syntax to the kinds of projects they’re best suited for. Understanding these differences can help you choose the right language based on your team’s goals, project complexity, and future scalability needs.
The Comparison of the Design Philosophy and Core Principles
Each language reflects a distinct philosophy that affects how developers approach problem-solving and software architecture.
Python emphasizes clarity and simplicity, guided by the “Zen of Python” — a set of principles that encourage readable, maintainable code. Its clean syntax supports rapid prototyping and makes it a strong choice for scripting, data analysis, and web development where frequent changes are common.
Java, by contrast, focuses on reliability, structure, and platform independence. The “Write Once, Run Anywhere” (WORA) principle, enabled by the Java Virtual Machine (JVM), allows Java applications to run across diverse systems. Because of this stability and efficiency, many companies hire Java developers for enterprise-grade systems, mobile platforms, and complex distributed networks that require robust performance under heavy load.
Syntax and Structure
The way a programming language handles syntax and structure can significantly affect development speed, code readability, and long-term maintenance.
Python’s dynamic typing means developers don’t need to declare variable types, which keeps the code clean and concise. Combined with its indentation-based syntax, Python encourages readable code — making it ideal for projects that require fast iterations and simple onboarding. However, this flexibility comes with the risk of runtime errors, so proper testing is essential.
Java, on the other hand, relies on static typing and a more verbose syntax. While that can slow down early development, it helps catch bugs at compile time and enforces a consistent code structure. For large-scale systems and long-term projects, this structured approach improves reliability and makes the codebase easier to manage over time.
Error Handling Approaches
The approach to handling code errors impacts the development speed and cost, reliability, data integrity, compliance, security, and other core features of business applications. That is why exploring this element within each programming language is essential.
Python relies on exceptions and try-except blocks to handle errors. If an error occurs, an exception is raised, allowing developers to control it and sustain the program’s flow.
When the Python interpreter encounters an error during runtime, it terminates with an automatic or customized error message unless it finds the try-except block that explains how to treat this exception.
Automatic error message:
>>> result = 10 / 0
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ZeroDivisionError: division by zero
Customized error message:
age = -1
if age < 0:
raise ValueError("Age cannot be negative")
The try block contains the exception(s) raising code, while the except block explains how to handle it (them), e.g., printing the error message or taking action:
try:
result = 10 / 0
except ZeroDivisionError as e:
print(f"Error occurred: {e}") # Signifying the error
try:
result = 10 / 0
except ZeroDivisionError:
result = 1 # Taking corrective action
print("Cannot divide by zero, defaulting result to 1")
The finally block will ensure the stated code execution regardless of whether the exception was raised or not. For example, it can close files or database and network connections, preventing resource leaks.
try:
file = open("example.txt", "r")
# Perform file operations
except IOError:
print("An I/O error occurred")
finally:
file.close()
print("File closed")
Java
Java employs structured exception handling with try-catch blocks, similar to Python. However, Java enforces explicit exception declarations, requiring developers to specify which exceptions to throw. This approach ensures that potential errors are documented and handled appropriately, promoting more rigorous error management.
In this example, several catch blocks identify the exceptions, then the program prints a message and continues:
public class MultipleCatchExample {
public static void main(String[] args) {
try {
int[] numbers = {1, 2, 3};
System.out.println(numbers[5]); // This will throw an ArrayIndexOutOfBoundsException
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Array index is out of bounds: " + e.getMessage());
} catch (ArithmeticException e) {
System.out.println("Arithmetic error: " + e.getMessage());
}
System.out.println("Program continues running");
}
}
In addition to try-catch blocks, Java provides a finally block that is executed regardless of the stated exceptions.
public class FinallyExample {
public static void main(String[] args) {
try {
int result = 10 / 0; // This will throw an ArithmeticException
} catch (ArithmeticException e) {
System.out.println("An error occurred: " + e.getMessage());
} finally {
System.out.println("This will always be executed");
}
System.out.println("Program continues running");
}
}
Java also differentiates between checked and unchecked exceptions. Checked exceptions require explicit handling using try-catch or declared with throws, while unchecked ones are ignored at compile time and don’t have to be explicitly handled.
Handling checked exceptions:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class CheckedExceptionExample {
public static void main(String[] args) {
try {
BufferedReader reader = new BufferedReader(new FileReader("example.txt"));
String line = reader.readLine();
System.out.println(line);
reader.close();
} catch (IOException e) {
System.out.println("An I/O error occurred: " + e.getMessage());
}
}
}
Handling unchecked exceptions:
public class UncheckedExceptionExample {
public static void main(String[] args) {
try {
int result = 10 / 0; // This will throw an ArithmeticException
} catch (ArithmeticException e) {
System.out.println("An arithmetic error occurred: " + e.getMessage());
}
}
}
When comparing Python vs Java, we see that they both utilize similar mechanisms for detecting, managing, and recovering from bugs. Python’s try-except blocks enable developers to catch and handle errors dynamically without predefined exceptions, making this language highly adaptable. Unlike Python, Java’s try-catch blocks enforce explicit exception declarations, requiring programmers to specify potential issues upfront, leading to more predictable error handling.
Java vs Python: Performance and Speed
The program execution time of a language impacts the optimization of computing resources, scalability of apps, real-time data processing, and the time of delivering services.
Let’s check these parameters for Java and Python.
Compilation vs Interpretation
How code is executed — whether it’s interpreted or compiled — has a direct effect on speed, development workflow, and scalability.
Python is an interpreted language, which means it processes code line by line at runtime. This typically results in slower execution compared to compiled languages like Java. But for developers, it also means quicker iterations — changes can be tested instantly, without recompilation, making Python a great fit for rapid prototyping and flexible development cycles.
Java code, on the other hand, is compiled into bytecode and then executed by the Java Virtual Machine (JVM). This extra compilation step pays off in performance: the JVM uses techniques like Just-In-Time (JIT) compilation to optimize runtime execution, making Java significantly faster and more resource-efficient, especially for high-load applications.
The Roles of the Java Virtual Machine and Python’s Interpreter
The runtime environment behind each language plays a key role in how applications perform, scale, and behave across platforms.
Java runs on the Java Virtual Machine (JVM), which allows compiled bytecode to be executed on any system with a JVM installed. This not only ensures portability but also enables performance improvements through features like adaptive compilation and automatic garbage collection — making Java a strong choice for scalable, high-load systems.
Python, on the other hand, uses an interpreter that reads and runs code line by line. While this simplifies development and debugging, especially during rapid prototyping, it can slow down execution for compute-intensive tasks. Still, for many use cases, Python’s ease of use and powerful libraries more than make up for the performance trade-offs.
Comparison of the Code Execution Speed
Java tends to deliver better performance than Python in computation-heavy tasks like image processing, large-scale simulations, or handling high-frequency financial transactions. Thanks to the Just-In-Time (JIT) compiler and efficient memory management in the Java Virtual Machine, Java can process large workloads with lower latency and better resource usage.
Python, while slower in raw execution, is often the preferred choice in AI and data science due to its specialized libraries like NumPy and TensorFlow. These libraries use highly optimized C and C++ backends, which help reduce the performance gap in many practical scenarios.
In the end, the right choice comes down to project priorities: if performance at scale is critical, Java might be the better fit. If fast experimentation, flexibility, and access to data science tools matter more — Python could be the smarter option.
Common Misconceptions Around Java and Python
Java and Python are two of the most widely used programming languages — but even with their popularity, some persistent myths continue to shape how businesses evaluate them. Before making a technology choice, it’s worth addressing a couple of common misconceptions that often mislead project teams.
Performance Myths
One common misconception is that Java performance surpasses Python’s. While Java’s compiled nature and JVM optimizations provide significant code execution advantages in computationally heavy tasks, this language doesn’t always win.
Python’s performance, particularly in data science and AI, benefits from optimized libraries like NumPy and TensorFlow, which leverage C and C++ for heavy-lifting computational tasks. Consequently, Python can be as efficient as Java in terms of AI and data analytics projects.
The difference in computational efficiency is more noticeable in large-scale systems or applications requiring intense computational power, where Java’s robustness shines.
Use Case Limitations
Another myth is that Python can be used only for scripting or web development, while Java is better for enterprise-level applications. In reality, both languages are highly versatile.
Python also excels in automation and building web applications, Data Science, ML, and even developing IoT systems. Java, known for enterprise applications, thrives in Android development, large-scale distributed systems, and high-performance computing.
Both languages offer extensive libraries and frameworks that enable them to adapt to a wide range of domains beyond their stereotypes.
Popular Libraries and Frameworks
Java and Python support a rich ecosystem of frameworks and libraries, including pre-built tools for streamlining the coding process. In this section, we’ll check the most popular and powerful tools for these languages and start with Python.
Domain | Top Python Libraries & Frameworks | Use Cases |
Web Development | Flask: A micro-framework offering simplicity and flexibility, making it ideal for development of smaller web applications and APIs Django: A high-level web framework that enables rapid building of secure and maintainable websites | Instagram: Django powers Instagram’s backend, enabling rapid engineering and scalability Pinterest: Utilizes Python for web app development, ensuring a seamless and responsive user experience |
Machine Learning | TensorFlow: Provides robust tools for building and training neural networks, making it essential for AI projects Scikit-learn: A library for ML offering simple and efficient tools for data mining and data analysis | Netflix: Uses Python for data analysis and ML to personalize recommendations and improve user experience Spotify: Leverages Python for data processing and ML to enhance music recommendations and user engagement |
Automation | Selenium: A tool for automating web browsers that is widely used for testing web applications PyAutoGUI: A library for programmatically controlling the mouse and keyboard used for automating tasks on the computer | Dropbox: Uses Python for backend services and automation, ensuring efficient file synchronization and storage NASA: Employs Python for scripting and automation in various space missions and research projects |
Big Data Processing | PySpark: The Python API for Apache Spark used for large-scale data processing Dask: A parallel computing library for analytics that is specifically designed for handling large-scale datasets | Airbnb: Uses Python extensively for analyzing large datasets, optimizing pricing strategies, and improving user experiences Google: Employs Python for various data processing tasks, including data analysis, machine learning, and system automation |
Java
Despite several disadvantages of Java, including memory consumption, verbose syntax, and garbage collection delays, this language remains a cornerstone in the world of software creation. Here are some of the most popular libraries, frameworks, and tools in the Java ecosystem, including their use cases.
Domain | Top Java Libraries & Frameworks | Use Cases |
Enterprise Applications | Spring Boot: Simplifies the creation of stand-alone, production-grade Spring-based applications Hibernate: An Object-Relational Mapping (ORM) library that simplifies database interactions by mapping Java objects to database tables | Amazon: Utilizes Java for building scalable and secure enterprise applications, including its e-commerce platform Goldman Sachs: Utilizes Java for developing trading platforms and financial analysis tools |
Messaging Systems | Apache Kafka: An open-source event streaming platform that is designed to handle high-throughput, low-latency messaging Apache Pulsar: A distributed messaging and streaming platform that supports both publish-subscribe and queue-based messaging models | Netflix: Uses Java to enable efficient content delivery to millions of users worldwide LinkedIn: Leverages Java to ensure seamless user interactions and data management for its global community of professionals |
Testing | JUnit: A unit testing framework that allows developers to write and run tests easily TestNG: A testing framework inspired by JUnit but with more powerful features | Google: Ensures the reliability and efficiency of its search engine and cloud services with Java-based testing frameworks Airbnb: Leverages Java for automated testing and continuous integration, ensuring the quality and stability of its platform’s features and functionalities |
Cloud Services | Quarkus: A Kubernetes-native Java stack tailored for OpenJDK HotSpot and GraalVM Micronaut: A modern, JVM-based framework for building modular, easily testable microservice and serverless applications | Amazon Web Services (AWS): Uses Java for developing and managing scalable and secure cloud computing services Google Cloud Platform (GCP): Leverages Java for building and optimizing its cloud-based infrastructure and services |
Using popular libraries, frameworks, and tools in Python and Java can significantly enhance your software engineering projects, ensuring security, scalability, and optimal task execution for diverse application areas. Sometimes, you might not even have to choose but to leverage the benefits of both languages, like Netflix.
Future Trends and Advancements
Java continues to adapt and thrive as technology evolves, just like Python does. Let’s explore the emerging trends and advancements that will shape the evolution of these two powerhouse programming languages.
Trends for Python
Python remains at the forefront of software development due to its broad range of applications and adaptability. Let’s examine the trends that have defined its evolution.
AI and Machine Learning: Python remains the go-to language for AI and ML projects due to its simplicity and extensive library support. Libraries like TensorFlow and PyTorch continue to evolve, offering faster and more efficient model training.
Cybersecurity and Ethical Hacking: Python is widely used in security analysis, penetration testing, and automation of security processes. Libraries like Scapy and PyCrypto help strengthen cybersecurity measures.
Automation and DevOps: Python is a powerful tool for automating repetitive tasks in building software, DevOps, and IT operations. It integrates with tools like Terraform and Ansible to automate cloud infrastructure management.
Web Development: Python’s frameworks like Django and Flask continue to be popular choices for web engineering, allowing businesses to create robust and scalable web applications.
Integration with Emerging Technologies: Python’s versatility allows it to integrate seamlessly with cutting-edge technologies like IoT and quantum computing, making it a valuable language for upcoming innovations.
Trends for Java
Let’s learn the latest trends shaping the evolution of Java, highlighting key developments and advancements that keep this language relevant and powerful.
AI and Machine Learning: Java’s strong emphasis on security and portability makes it a popular choice for AI and ML applications. Libraries like Deeplearning4j and RapidMiner are gaining popularity.
Microservices Architecture: Java frameworks like Spring Boot and Eclipse MicroProfile simplify the creation and deployment of microservices, enabling flexible and scalable applications.
Cloud Computing and Serverless Architecture: Java’s platform independence and scalability make it ideal for cloud computing environments. It supports seamless operation across various systems and hardware configurations.
DevOps Integration: Java’s strong tooling support and large ecosystem make it well-suited for DevOps practices, improving the efficiency of software delivery processes.
Enhanced Concurrency and Performance: Projects like Loom and Panama enhance Java’s concurrency and foreign function interfacing capabilities, making it more performant for modern applications.
As an offshore/nearshore tech talent provider, we can confirm businesses select these programming languages for their software creation projects. Our statistics show that Mobilunity’s clients hire nearly 2.5 times more remote Java engineers than Python experts for back-end development.
Comparison of Python vs Java Engineers’ Salaries
Stack Overflow shows that in 2024, the average annual salary of a Python developer equaled $68,000 and Java’s — $61,000 (globally). Below, we’ll estimate the approximate cost of hiring these developers in popular offshore and onshore locations according to talent.com and DOU.
Costs for developers based in Latin America, Asia, and Eastern Europe are on average 30%-50% lower than in the USA or Western Europe.
This is achievable due to lower living costs and local hiring expenses. However, developers based in Ukraine, Poland, and the Baltic countries can be a more suitable option due to their strong backgrounds, business-focused work attitude, and cultural fit.
Our partners successfully launch their own products with our dedicated development teams based in Ukraine.
For instance, a Canadian agency, 3e Joueur, turned to us after not quite favorable cooperation with a few tech team providers. They needed a seasoned Python engineer with expertise in banking. Our recruiters vetted highly qualified candidates from Ukraine and onboarded one of them in just two weeks.
In three years, the company decided to launch its own fintech product, FinX. It asked for their team extension to 5-6 people while offering the CTO role to the first developer.
Python versus Java: Decision-Making Framework
Choosing Python over Java or vice versa can be challenging. Here’s a mini guide to help you make an informed decision based on your project’s needs.
Questions | Suitable Language |
Q1. What is your project type? | |
Web Application Development | Python’s Django & Flask frameworks are excellent for rapid development, while Java’s Spring Boot framework is suitable for applications that require robustness and scalability |
Data Analysis | Python is the preferred choice for data analysis and machine learning, thanks to libraries like NumPy, Pandas, and TensorFlow |
Enterprise Applications | Java is the go-to language for large-scale enterprise applications, offering stability and performance |
Q2. What are your performance needs? | |
Real-time processing | Java’s compiled nature and JVM optimizations make it more appropriate for real-time processing tasks |
Scalability | Java’s strong concurrency support and scalability make it ideal for applications with high-performance demands |
Q3. What development speed do you need? | |
Prototyping | Python’s simplicity and dynamic typing allow for rapid prototyping and software engineering, making it ideal for projects that need quick iterations |
Long-term projects | Python’s versatility, extensive libraries, and strong community support make it an excellent choice for long-term projects in various domains. Java’s static typing and compile-time error checking make it a more suitable choice for long-term projects that require maintainability and robustness |
Q4. What is your team’s expertise? | |
Learning curves | Python’s syntax is easier for beginners, making it a good choice for teams with less experience. Java’s verbose syntax requires more initial learning but can pay off in long-term projects. |
By evaluating these factors, you can select the language that aligns with your project’s requirements and decide which one is better suited for your goals.
Wrapping Up
While Python is more suited for AI, automation tasks, and fast development, organizations prefer Java for large-scale, high-capacity enterprise apps. Both languages continue to evolve, remaining essential tools for modern software development.By understanding each language’s strengths and limitations, businesses can select the technology that better suits their project scope, functional requirements, and team expertise. Companies can build scalable, maintainable, optimized applications with the right tech stack or benefit from both languages. And with the right IT staffing partner they can find top-tier Python or Java developers to do that.